Simple Mqtt Client
Overview
simplemqttclient.c is a client program that mimics a sensor reading from an IoT device that can read metrics like sound and light; it uses the libmosquitto library.
Certain configuration settings can be set for initializing a mosquitto publishing client to a server. These settings can be found at the top of mqttclient/simplemqttclient.c. The configurations are set for mosquitto communication on a localhost mosquitto server. Mosquitto, like Kafka, sends messages to special channels called topics that other mosquitto servers can subscribe to. It is this topic that mosquitto and Kafka will share, with the help of a connector program, to share their messages.
// Defaut Mosquitto Server settings #define MQTT_HOSTNAME "localhost" #define MQTT_PORT 1883 #define MQTT_USERNAME "admin" #define MQTT_PASSWORD "admin" #define MQTT_TOPIC "test"
Creating and Connecting client to Mosquitto Server
Establishing mqtt client(simplemqttclient.c)mosquitto_lib_init() Struct mosquitto* mosq = mosquitto_new(NULL,true,NULL); mosquitto_connect(mosq.MQTT_HOSTNAME,MQTT_PORT,0);
- Line 3: mosquitto-client, the mosquitto-library, and a connection to localhost
Publishing to the Mosquitto Server / Subscriber
From there, the client will create a formatted JSON-string that mosquitto will use as its message that can be converted to a sensor-object by Kafka later on
Publish to Mosquitto(simplemqttclient.c)char *text = (char *)malloc(sizeof(char)*128); sprintf(text, “{ light : %f. sound : %f }” , metric1, metric2 ); mosquitto_publish(mosq, NULL, MQTT_TOPIC, strlen(text), text, 0, false);
Also note that multiple mosquitto-clients can run and publish messages to one mosquitto server simultaneously. This is an important function to take note of as this means, in later parts of the project, that many clients can run either on a single machine or on multiple machines and feed its messages to other programs.
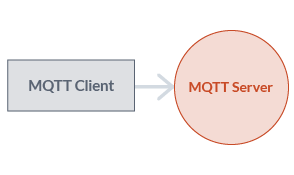
Source Code
Complete source code used for the GridDB Kafka and MQTT Application can be downloaded from the following:
Download: griddb-kafka-mqtt-application.tar.gz