初期コンテナの作成
データ生成クライアントのデータフロー
データ生成クライアントは、以下の5つのCプログラムによって作成された実行ファイルを利用します。
- registerCoffeeChain.c
- insertCoffeeStores.c
- insertCoffeeMachines.c
- preloadRecords.c
- generateEspressoRecords.c
これらのアプリケーションの多くは、このCファイルに記載されているメソッドも使用します。
gridstorefunctions.h
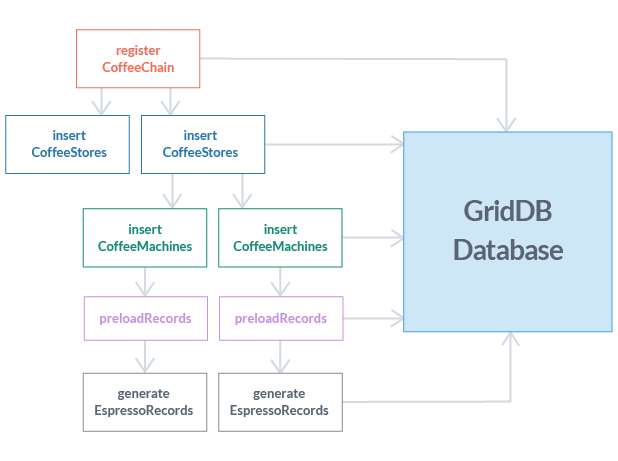
gridstorefunctions.h
クライアントスクリプトで使用される5つの実行可能ファイルはすべて、gridstorefunctons.cで定義されたメソッド呼び出しおよび参照を行います。 これらのメソッドの多くは、ランダムなデータの作成やコンテナの作成、GridDBの更新に関連しています。 gridstorefunctions.hには、HOST, PORT, CLUSTER_NAME, USER, PASSWORD
の5つの定数があります。 別のGridDBクラスタに接続する場合は、希望の値に設定してください。
// Change to the cluster configuration you wish to connect to, this is the default #define HOST "239.0.0.1" #define PORT "31999" #define CLUSTER_NAME "defaultCluster" #define USER "admin" #define PASSWORD "admin"
registerCoffeeChain
このプログラムは、コーヒーチェーンコンテナの名前である単一のコマンドライン引数をとり、それをGridDBに挿入します。
GSGridStore* gridstore = makeGridStore(HOST,PORT,CLUSTER_NAME,USER,PASSWORD);
これは、gridstorefunctions.h
にある単純にGridDBへの接続を確立するメソッドを使います。
GSCollection* collection = makeCoffeeStoreCollection(gridstore,argv[1]);
これにより、Coffee Chainコンテナが作成されてGridDBに挿入されます。 このアプリケーションでは、コンテナはstructではなくcontainerInfoオブジェクトでより一般的にまたは動的に作成されました。
GSCollection* coffeeChain; GSColumnInfo columnInfo = GS_COLUMN_INFO_INITIALIZER; …. // Create Column Schema columnInfo.name = "store_id"; // Primary key of coffee stores is a 'store_id' columnInfo.type = GS_TYPE_STRING; …. // Address Column columnInfo.name = "address"; // All stores have an 'address' location columnInfo.type = GS_TYPE_STRING; …. // Latitude Column columnInfo.name = "latitude"; // Stores also can be identified as (latitude and longitude) columnInfo.type = GS_TYPE_FLOAT; …. // Longitude Column columnInfo.name = "longitude"; columnInfo.type = GS_TYPE_FLOAT;
上記のように、作成されたスキーマは上記のスキーマの図と似ています。 緯度と経度は精度のためにfloat にキャストされます。 構造体を持たない列スキーマを作成するには、containerInfo オブジェクト
とcolumnInfo 配列
が必要です。 配列内の各columnInfo
には、列の名前と型があります。containerInfo
には、すべての列スキーマ、コンテナ名、その行キー、およびそのコンテナのタイプが保持されます。 これらのオブジェクトとそのプロパティがすべて設定されると、コンテナを作成してgsPutCollectionGeneral
メソッドを使用してGridDBに挿入できます。
このコンテナが作成されると、そのコンテナ名を使用して後でコーヒーストアを挿入できます。
insertCoffeeStores.c
このプログラムは、次のコマンドライン引数をとります。
- The name of the coffee chain it belongs to
- The store id of the coffee store
- The address of the coffee store
- The latitude of the coffee store
- The longitude of the coffee store
プログラムは、コーヒーストアが所属するコーヒーチェーンコンテナを取得することから始まります
gsGetCollectionGeneral(gridstore,argv[1],&coffeeStore);
次に、プログラムは、コーヒーチェーンにコーヒーストアを表す行を挿入するために、これらの引数を受け取り、解析します。
insertCoffeeStore(coffeeChain,argv[2],reformatAddress(argv[3]),latitude,longitude);
gsSetRowFieldByString(storeEntry,0,store_id); // Set store's id gsSetRowFieldByString(storeEntry,1,address); // Set store's addresss gsSetRowFieldByFloat(storeEntry,2,latitude); // Set store's latitude gsSetRowFieldByFloat(storeEntry,3,longitude) gsPutRow(coffeeChain,NULL,storeEntry,NULL);
ここで、行フィールドが関数に渡されたコマンドライン引数によって設定され、行はコーヒーチェーンに挿入されます。 コーヒーストアはコーヒーチェーンに属しているだけでなく、コーヒーマシン用のコンテナでもあります。 そのため、機械(machines) を保管するためのコーヒーストアコンテナも必要です。
makeMachineCollection(gridstore,argv[2]);
コンテナを作成する方法は、makeCoffeeStoreCollection
関数に似ています。 このコレクションには、マシンのインストール日を保持するtimestamp
列があります。 マシンがインストールされてからのサイクル数を保持するinteger
列もあります。 最後のクリーンサイクル以降のサイクルを保持する列もあります。 マシンのクリーンサイクルが開始されると、その列の値はゼロにリセットされます。
insertCoffeeMachines.c
このプログラムは、これらのコマンドライン引数を取り、コーヒーマシンコンテナを作成します。
- The store id of the coffee store the machine belongs to
- The serial number of the coffee machine
- The model number of the coffee machine
- The installation date of the coffee machine as a timestamp string
新しいコーヒーマシンの場合、machine がレコードの生成を開始していないため、cycle_new
およびcycles_clean
の値として0が設定され、最初に列としてコーヒーマシン(cofee machine) に挿入されます。
まず、コーヒーマシンが属するコーヒーストアコンテナを入手します。
gsGetCollectionGeneral(gridstore,argv[1],&coffeeStore);
これでコーヒーマシンは、gridstorefunctions.c
ファイルのinsertCoffeeMachines
関数の行としてコーヒーストアに挿入できます。
insertCoffeeMachines(coffeeStore,argv[2],argv[3],timestamp,0,0);
gsSetRowFieldByString(coffeeMachine,0,serial_number); gsSetRowFieldByString(coffeeMachine,1,model_number); gsSetRowFieldByTimestamp(coffeeMachine,2,installation_date); gsSetRowFieldByInteger(coffeeMachine,3,cycles_new); gsSetRowFieldByInteger(coffeeMachine,4,cycles_clean); gsPutRow(coffeeStore,NULL,coffeeMachine,NULL);
コーヒーマシンがすでに存在する場合、プログラムは直ちに終了します。
Source Code
以下のリンクから、データ生成クライアントおよびデータ視覚化コンポーネントのアプリケーションおよびそのソースコードをダウンロードできます。
Download: datavisualisation_application.tar.gz